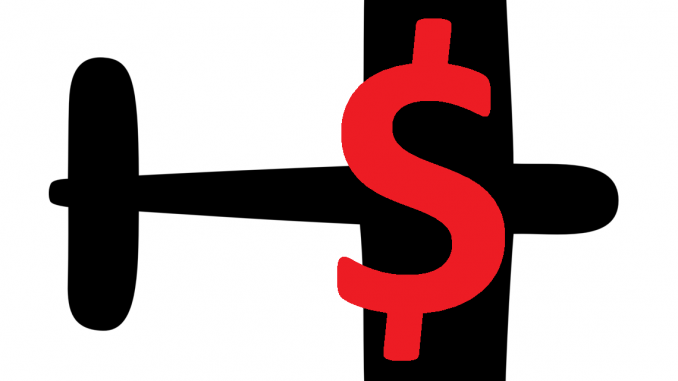
Recently I was asked how to find the cheapest flights via JavaScript to use it on a website. The best possibility I found was the Google QPX API, which is the API behind Google Flights.
Online many people have done this, but almost all of them used JQuery for the POST request. This script was designed to be simple and easy, thus, it works without additional libraries.
The script does a POST request to the QPX API and receives much data back. So far it only examines the cheapest flight option, but can easily be changed to compute other measures.
To get this working you need to have your own API key, which is free for up to 50 requests per day. You can make one here and choose “Browser Key”. Insert it in “MY_API_KEY” in the code below. If you want to change the computed measures, you might want to have a look at the QPX API.
Hopefully this might help someone with a similar problem!
/*
#==========================================#
# PapeCoding #
# Questions to www.PapeCoding.de #
#==========================================#
*/
var defaultFlightRequest = {
"request": {
"slice": [
{
"origin": "FRA",
"destination": "EAS",
"date": "2016-12-11"
}
],
"passengers": {
"adultCount": 1,
"infantInLapCount": 0,
"infantInSeatCount": 0,
"childCount": 0,
"seniorCount": 0
},
"solutions": 20,
"saleCountry": "DE",
"refundable": false
}
};
//Test Call like this
checkFlightMinPrice("FRA", "EAS", "2016-04-26", mySuccessFunction)
//or like this one:
/*
checkFlightMinPrice("FRA", "LCY", "2016-04-26", function(price, currency){
//Do Stuff here
alert("The Currency is " + currency);
alert("The minimal price is " + price);
});
*/
function checkFlightMinPrice(origin, destination, date, callBack){
var xhttp;
if (window.XMLHttpRequest) {
xhttp = new XMLHttpRequest();
} else {
// code for IE6, IE5
xhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
xhttp.open('POST', 'https://www.googleapis.com/qpxExpress/v1/trips/search?key=MY-API-KEY', true);
xhttp.setRequestHeader('Content-Type', 'application/json; charset=utf-8');
//modify default flight request to match our search
var myFlightRequest = defaultFlightRequest;
myFlightRequest.request.slice[0].origin = origin;
myFlightRequest.request.slice[0].destination = destination;
myFlightRequest.request.slice[0].date = date;
xhttp.send(JSON.stringify(myFlightRequest));
xhttp.onreadystatechange = function() {//Call a function when the state changes
if(xhttp.readyState == 4){ //answer is ready
switch(xhttp.status == 200) {
case 200: //Success
var minPrice = Number.MAX_SAFE_INTEGER;
var allTrips = JSON.parse(xhttp.response).trips;
var numTripOptions = allTrips.tripOption.length;
var currency = allTrips.tripOption[0].pricing[0].saleTotal.slice(0,3);
for(var i = 0; i < numTripOptions; i++){
var numPricingOptions = allTrips.tripOption[i].pricing.length;
//determine smallest price for this option
for(var j = 0; j < numPricingOptions; j++){
var priceEUR = Number.parseFloat(allTrips.tripOption[i].pricing[j].saleTotal.slice(3));
if(priceEUR < minPrice) minPrice = priceEUR;
}
}
callBack(minPrice, currency);
break;
case 404: //Page not found (broke the url?)
alert("Error: " + xhttp.responseText);
break;
case 403: //Forbidden or keys not valid (maybe the all calls for this day are made?)
//do some default stuff here
break;
case 500: //Server error (you are screwed)
alert("Error: " + xhttp.responseText);
break;
}
}
}
}
function mySuccessFunction(price, currency){
alert("The minimum price is: " + price + " " + currency);
}
Leave a Reply